스프링부트에서 의존성을 추가하고 싶을 때 주로 스프링부트에서 제공하는 starter 라이브러리를 의존성 설정파일에 추가한다.
예를들어 Mybatis 라이브러리를 추가하고자 하면 제공되는 starter 라이브러리를 의존성설정파일(메이븐의 경우 pom.xml)에 아래처럼 추가한다. starter 라이브러리는 단순히 의존성만 추가되는 것이 아니라 자동설정을 포함 하는데, 이것에 대해 자세히 알아본다.
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
mybatis starter 라이브러리를 기준으로 포스팅할 예정이며 다른 라이브러리도 비슷하다.
mybatis-spring-boot-starter
스프링부트 프로젝트의 pom.xml에 추가한 mybatis-spring-boot-starter 자체에는 많은 파일이 있지는 않다.
META-INF 폴더에 pom.xml과 pom.properties 파일만 있는 정도이다.
아래는 mybatis-spring-boot-starter의 파일구성과 pom.xml이다. starter의 pom.xml에는 사용될 다른 라이브러리와 autoconfigure(mybatis-spring-boot-autoconfigure) 라이브러리가 포함되어 있는 것을 볼 수 있다.
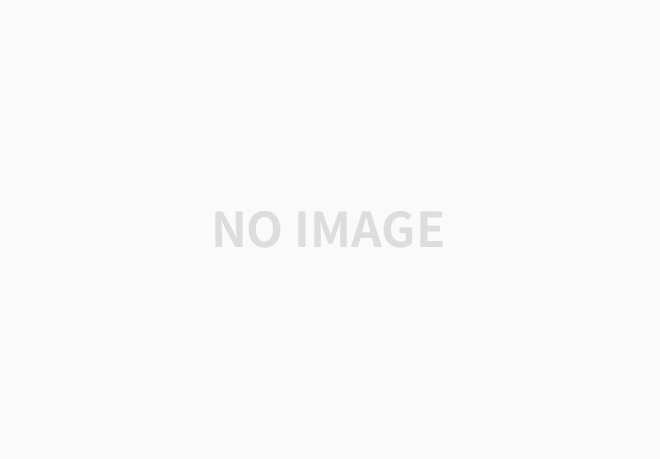
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot</artifactId>
<version>2.1.1</version>
</parent>
<artifactId>mybatis-spring-boot-starter</artifactId>
<name>mybatis-spring-boot-starter</name>
<properties>
<module.name>org.mybatis.spring.boot.starter</module.name>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-autoconfigure</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
</dependency>
</dependencies>
</project>
mybatis-spring-boot-autoconfigure
pom.xml 중간에 추가된 autoconfigure는 자동설정 클래스를 포함하고 있다.(spring.factories 및 XxxAutoConfiguration.class 포함)
스프링부트 프로젝트 기동시 메인 클래스의 @EnableAutoConfiguration 애노테이션이 spring.factories 파일을 읽어들여 자동설정을 진행하는데 자세한 내용은 이전 포스팅을 참고하자.
이전 포스팅 링크 :
2020/12/01 - [Dev/스프링부트] - @SpringBootApplication에 대해서(Springboot 기본구조)
@SpringBootApplication에 대해서(Springboot 기본구조)
스프링부트 기본 프로젝트를 생성하면 main메서드를 포함하는 시작 클래스가 있고, 이 클래스에는 @SpringBootApplication이 마킹되어 있다. @SpringBootApplication는 다수의 애노테이션으로 이루어진 메타
yangbox.tistory.com
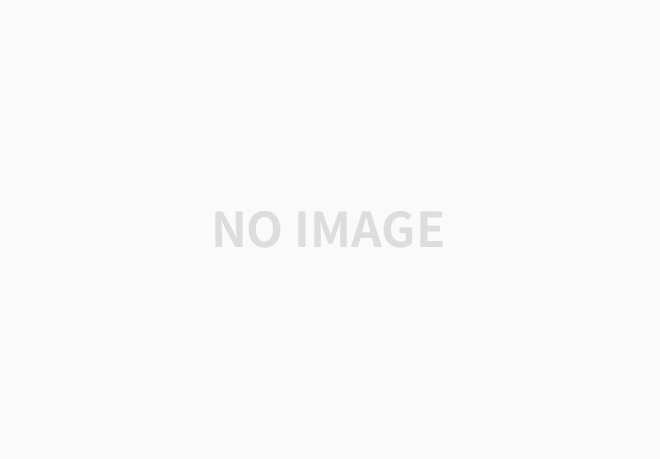
mybatis-spring-boot-autoconfigure의 내용(위 사진)을 보면 MybatisAutoConfiguration 자동설정클래스도 보이고, MybatisProperties 프로퍼티 클래스도 보인다. 이 중 프로퍼티 클래스에 대해 좀 더 살펴보면 보통 어플리케이션에 프로퍼티 설정을 위해 application.yml, application.properties와 같은 파일을 두고 키-값으로 프로퍼티를 작성한다. 이 값들을 스프링부트가 읽어 들여 변수에 세팅하는 클래스가 MybatisProperties 같은 프로퍼티 클래스이다.
좀 더 자세히 보자. MybatisProperties의 소스코드 일부이다. @ConfigurationProperties는 외부 프로퍼티를 사용하는 클래스라는 의미이고 prefix값을 지정할 수 있다. 예를들어 application.properties에서 'mybatis'로 시작하는 프로퍼티를 작성하면 읽어 들여 클래스의 변수에 바인딩된다.
mybatis.configLocation 프로퍼티 작성 -> MybatisProperties 클래스의 configLocation 변수에 바인딩
@ConfigurationProperties(prefix = MybatisProperties.MYBATIS_PREFIX)
public class MybatisProperties {
public static final String MYBATIS_PREFIX = "mybatis";
private String configLocation; // 프로퍼티 설정파일에서 값을 읽어들여 바인딩된다.
// 생략
}
변수가 바인딩된 프로퍼티 클래스를 AutoConfiguration 자동설정 클래스에서 @EnableConfigurationProperties(MybatisProperties.class) 애노테이션을 마킹하여 바인딩된 변수(프로퍼티 설정파일의 값)를 이용하여 자동설정 한다.
@org.springframework.context.annotation.Configuration
@ConditionalOnClass({ SqlSessionFactory.class, SqlSessionFactoryBean.class })
@ConditionalOnSingleCandidate(DataSource.class)
@EnableConfigurationProperties(MybatisProperties.class)
@AutoConfigureAfter({ DataSourceAutoConfiguration.class, MybatisLanguageDriverAutoConfiguration.class })
public class MybatisAutoConfiguration implements InitializingBean {
private static final Logger logger = LoggerFactory.getLogger(MybatisAutoConfiguration.class);
private final MybatisProperties properties; // 바인딩된 properties
// 생략
}
요약
스프링부트에서 의존성을 추가하고 싶다면 제공되는 stater가 있는지 알아본다.
starter는 단순히 라이브러리를 추가하는 것에 더해서 autoconfigure 라이브러리를 참조한다.
autoconfigure의 spring.factories에 정의된 자동설정 클래스들이 실행되고 스트링부트 프로젝트의 application.properties 같은 프로퍼티 설정파일에서 값을 읽어 들여 자동설정에 사용한다.
'개발 > Spring & Spring Boot' 카테고리의 다른 글
ApplicationRunner, CommandLineRunner (0) | 2020.12.02 |
---|---|
SpringApplication(FailureAnalyzers, Application Events and Listeners, WebApplicationType) (0) | 2020.12.02 |
@SpringBootApplication에 대해서(Springboot 기본구조) (0) | 2020.12.01 |
Spring Core Technologies 4 (0) | 2019.09.16 |
Spring Core Technologies 3(Resource, ResourceLoader) (0) | 2019.09.15 |